Collisions 17/03/24
Figured out some of the issues from last week, it's still a super rough system but it'll work in the right narrow context. Due to the structure of the engine (designed to process game objects in parallel), calling a collision test from one game object's update() function, will only be able to test against the previous frame's collision. That works fine when testing against static objects, though:
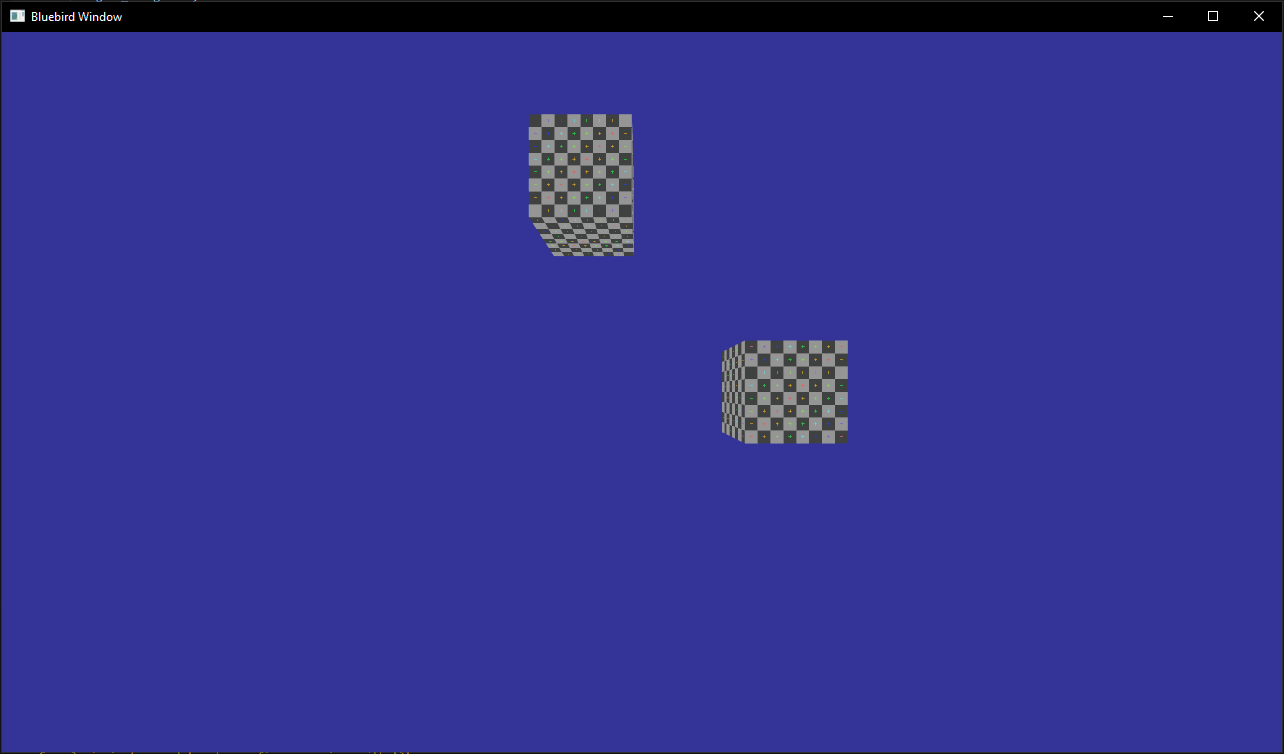
The system could be expanded to automatically call collision tests after each frame, so collisions between 2 moving objects can be handled more accurately. It's not something I'm looking into immediately though, next week is looking like time to patch some important holes - stuff like passing a delta time to game objects, or retrieving read-only game object references, or cleaning up public struct fields into private fields with public getters. Lots of important odd jobs. See you then! - Chill (:>{ ¦
Slow Progress... 11/03/24
It's late too, sorry! I found more problems than solutions this week so I don't have anything to show :(
I was working on collision detection last week. Rendering and structuring game objects are both things I've played around with before, but I've never written collision checks before, aside from some simple 2D checks in PICO-8. 3D shapes aren't the scary thing here though, the real enemy is 3D transformations - a cube vs cube test is super easy if both are axis-aligned, but things get complicated when we throw rotations (or worse, scale and rotation) into the mix. Likewise I can write a sphere vs sphere test, but an ellipsoid would be new. I think the theory would be to model the ellipsoids mathematically, set the formulae equal to eath other and derive the code from that, but man it sounds like a pain. And I know how to modify the equations to change the ellipsoid's center or scale, but I wouldn't have a clue how to apply a 4x4 transformation matrix to it...
To avoid all that trouble, my solution is to check whether we can do the "easy" check - i.e. for the cube vs cube test, the engine checks if both cubes are axis aligned. If they are, we can do the easy test that I know how to implement, and if they aren't we just crash, haha. That's a problem for future me, but it allows me to check the rest of the system is working. More on that system next time, though! - Chill (:>{ ¦
Animated Sprites 03/03/24
2nd Devlog, this week I've been working on Sprites. The concept is really simple, every Sprite uses the "quad" primitive Mesh, scales itself up in the world such that 1 pixel becomes 1 world unit, and scales down its texture coordinates to select a sprite from a larger spritesheet texture like this one:
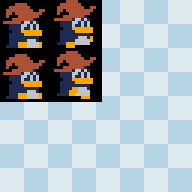
There were actually a lot of problems implementing this in a way I was happy with, working around the rest of the code and undoing some bad assumptions I'd made earlier in the project. You'd think a Sprite resource should contain a reference to the Texture resource it uses, but I wanted Sprites able to be assigned an arbitrary Material, and materials define what texture resource to use... so these Sprite resources just define coordinates, and apply those coordinates to whatever texture is being drawn.
This builds off the proto-component system, adding a SpriteInstance to the prior MeshInstance. There's some room to refine the system but I'm really happy with this design :)
Here's the sprite sheet from earlier in action:
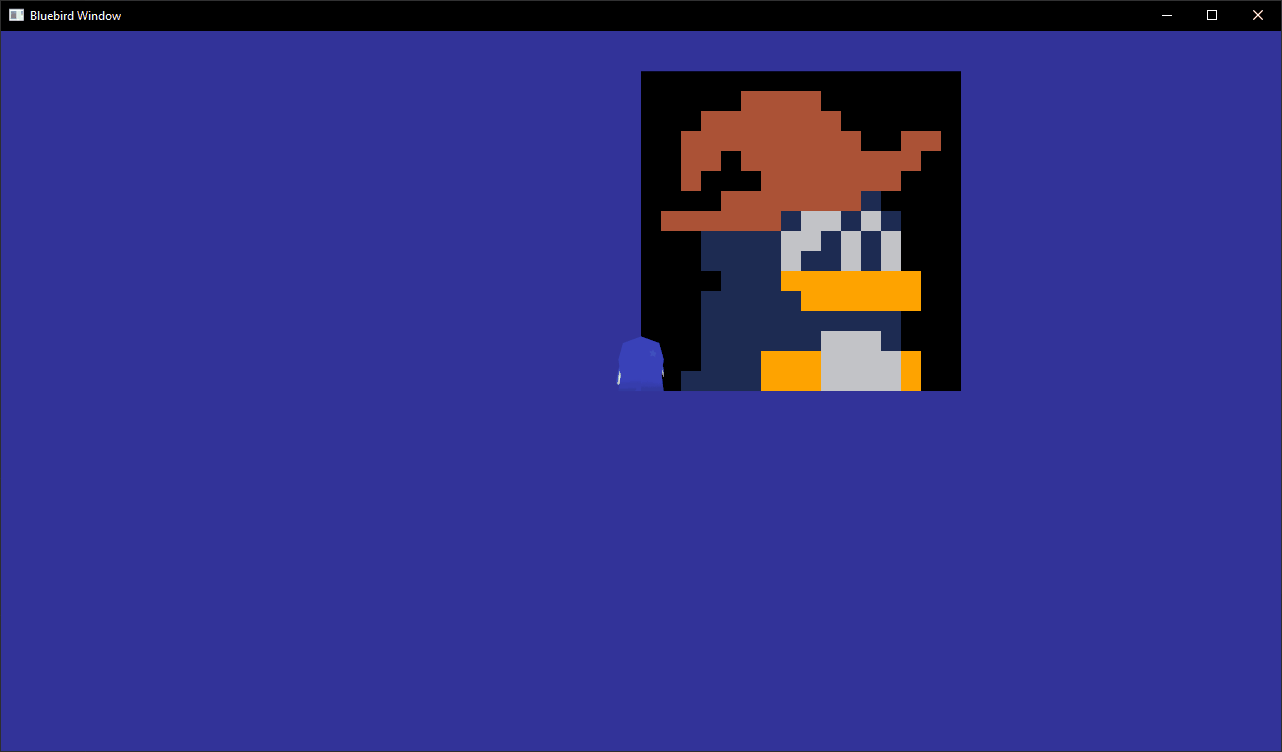
There are still some fundamental pieces to build but I might start work on a test project, like a little gamejam-size project to make sure core functionality works in a practical environment. Trying to avoid making a space shooter, they're great test projects but I've made plenty already! Trying to come up with something a bit more exciting. - Chill (:>{ ¦
First Update 25/02/24
This is the first update but far from the first week of development - I started work sometime late last year, took a break, and picked it up with a much more stable schedule recently.
Because of all that, this week I'm just going to outline the current state, and then subsequent logs can talk about weekly progress.
So, this project is some kind of game engine/framework, written in Rust, with minimal dependencies on other crates. It'd be awesome to make games with it, but I'm also making it to practice Rust, as well as to learn how to build this kind of system, and also because it's just a fun project to make :)
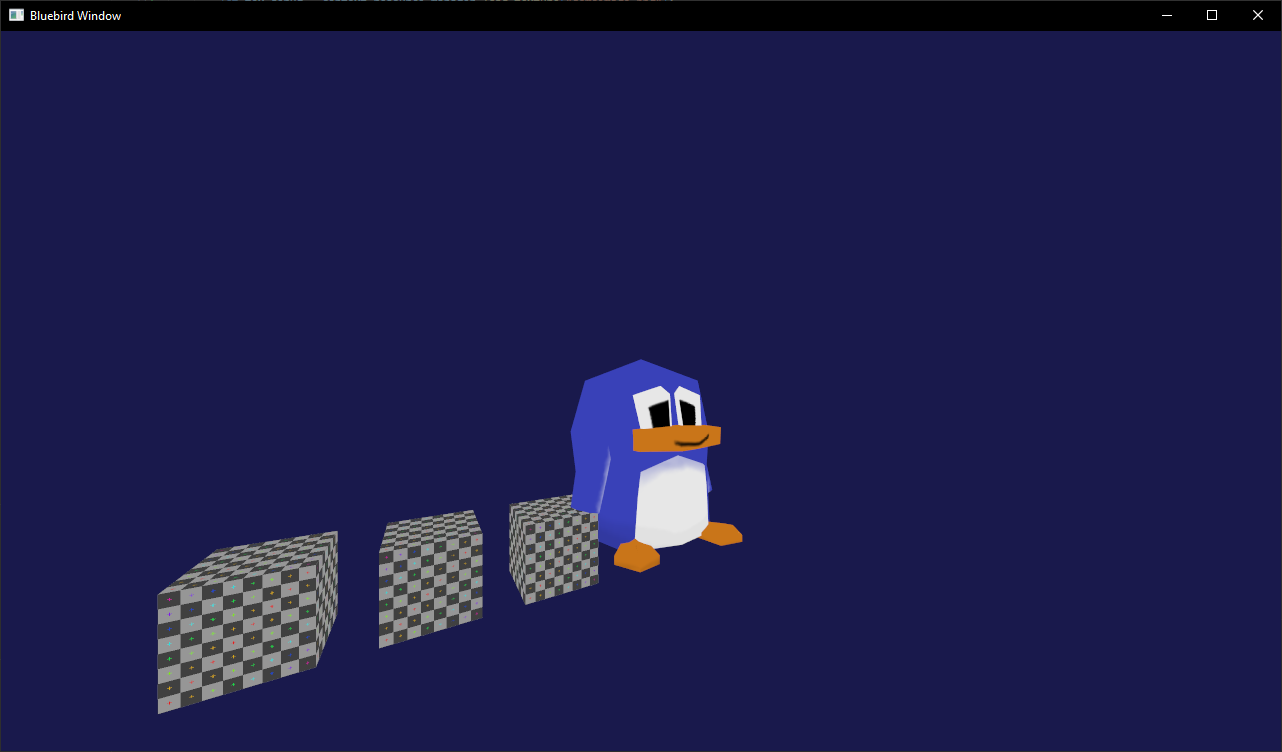
Here it is, demonstrating Texture and Mesh loading. The Actor struct in this design has a generic Script member, which the programmer can implement to give Actors behaviour. Before this project I almost exclusively used C++, but Rust is much more strict with references and mutable access. My design is to give a Script mutable access to itself and its Actor during its per-frame Update() function, as well as a reference to the previous frame's entire Scene, containing all Actors. This should make it possible to update Actors simultaneously across multiple threads, as well as cooperating with Rust's memory safety. This isn't perfect as there will be situations where Actor/Script order matters, so there's a generic GameManager the programmer can implement like a Script, to run behaviour before or after all Scripts (i.e. for a Camera following a player).
My recent work has been around asset loading - took a while and a lot of silly mistakes to get meshes and textures rendering correctly, but after that is was mostly smooth sailing refactoring hacky code into a really clean ResourceManager system. Really proud with how this turned out, it's consise to use but reliable. The main technical hurdle was building a system where resources can be 'unavailable', which would allow for resources to be loaded asynchronously in the future. For example, "load_mesh" doesn't actually load the Mesh, but pushes the filename to be loaded later, when the OpenGL context is available.
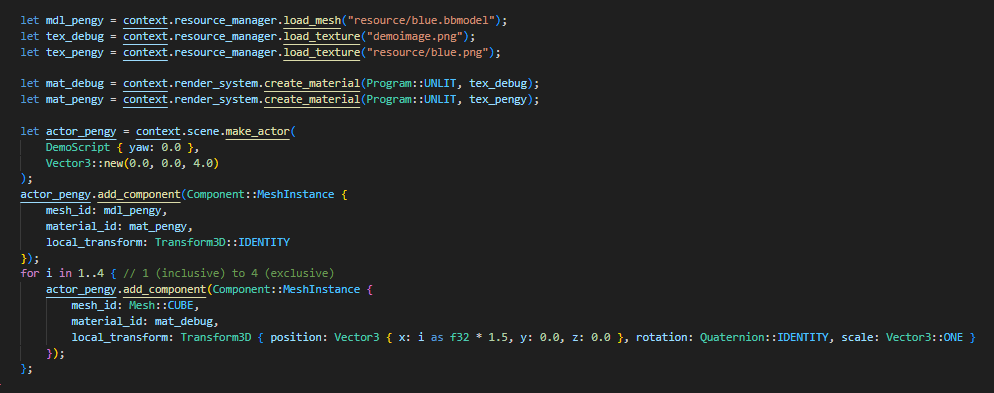
This is the code to generate the above scene (omitting Pengy's movement code). Actors used to have an option of 0 or 1 Meshes and Materials, the most recent addition is that proto-component system, which currently is just a list of Mesh Instances but I'll be expanding soon. These Components could be sprites, colliders, lights... some of those might show up in these dev logs soon :)
If you got this far, thanks so much for reading my ramblings!! Consider following to see more next week! - Chill (:>{ ¦